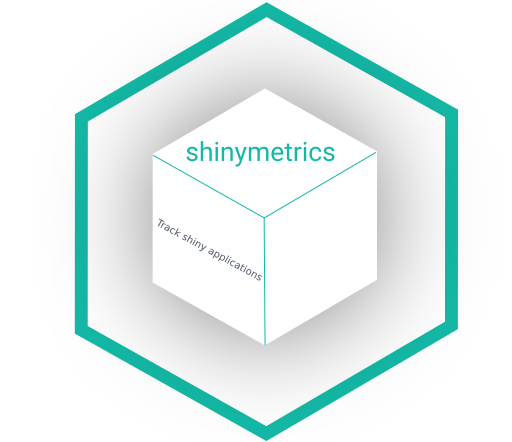
Consent
consent.Rmd
These prompts toggle tracking and cookies on and off but DO NOT store consent, if that is required you must implemented it yourself.
Collect Consent
There are two ways to collect user consent to initialise tracking:
- Use one of provided UI functions to prompt the user for consent
- Build something custom
Pre-built Prompts
:+1: It is recommended to use the pre-built prompts.
Pre-built prompts to place in your UI, use depends on style preference and Bootstrap version, all their arguments are optional.
-
trackingToastBS5()
- Bootstrap 5 notification -
trackingModalBS5()
- Bootstrap 5 modal -
trackingModalBS4()
- Bootstrap 4 modal -
trackingModalBS3()
- Bootstrap 3 modal
library(shiny)
library(shinymetrics)
tracker <- Shinymetrics$new()$track_recommended()
ui <- fluidPage(
theme = bslib::bs_theme(version = 5L),
tracker$include(),
trackingToastBS5(
full_width = TRUE,
background = "white",
position = "bottom",
prompt = "Please enable cookie tracking :)",
accept = "Yes, sure",
reject = "No!"
),
div(
style = "min-height:100vh;",
h1("Prompt V5")
)
)
server <- function(input, output, session) {
shinymetrics_server()
}
shinyApp(ui, server)
Custom Prompt
Alternatively you can of course create a custom prompt of your choosing.
library(shiny)
library(shinymetrics)
tracker <- Shinymetrics$new()$track_recommended()
ui <- fluidPage(
tracker$include()
)
server <- function(input, output, session) {
shinymetrics_server()
observe({
# shinymetrics tracking is already enabled
# no need to show the modal
if(is.null(input$shinymetricsEnabled))
return()
if(input$shinymetricsEnabled)
return()
showModal(
modalDialog(
title = "Tracking",
p("Enable tracking?"),
actionButton("accept", "Accept"),
actionButton("reject", "Reject"),
easyClose = FALSE,
footer = NULL
)
)
})
observeEvent(input$accept, {
removeModal()
shinymetrics_enable()
})
observeEvent(input$reject, {
removeModal()
shinymetrics_disable()
})
}
shinyApp(ui, server)
Cookies and testing
When shinymetrics is enabled it stores some information as cookie so the user does not have to enable tracking at every visit.
This means that once you have enabled tracking you have cookie stored in your browser and the consent prompt will no longer show. This may not be convenient while testing or building a cutom prompt. There are two ways to tackle this issue:
- Visit your application in “incognito” mode so it does not store cookies. See instructions for Chrome, Firefox, Safari, and Edge
- From your browser clear the cookies for your app. Visit your application in your browser then, generally, click on the icons left of the left of the URL and clear the cookies.